How to Increment and Decrement Variables in Bash
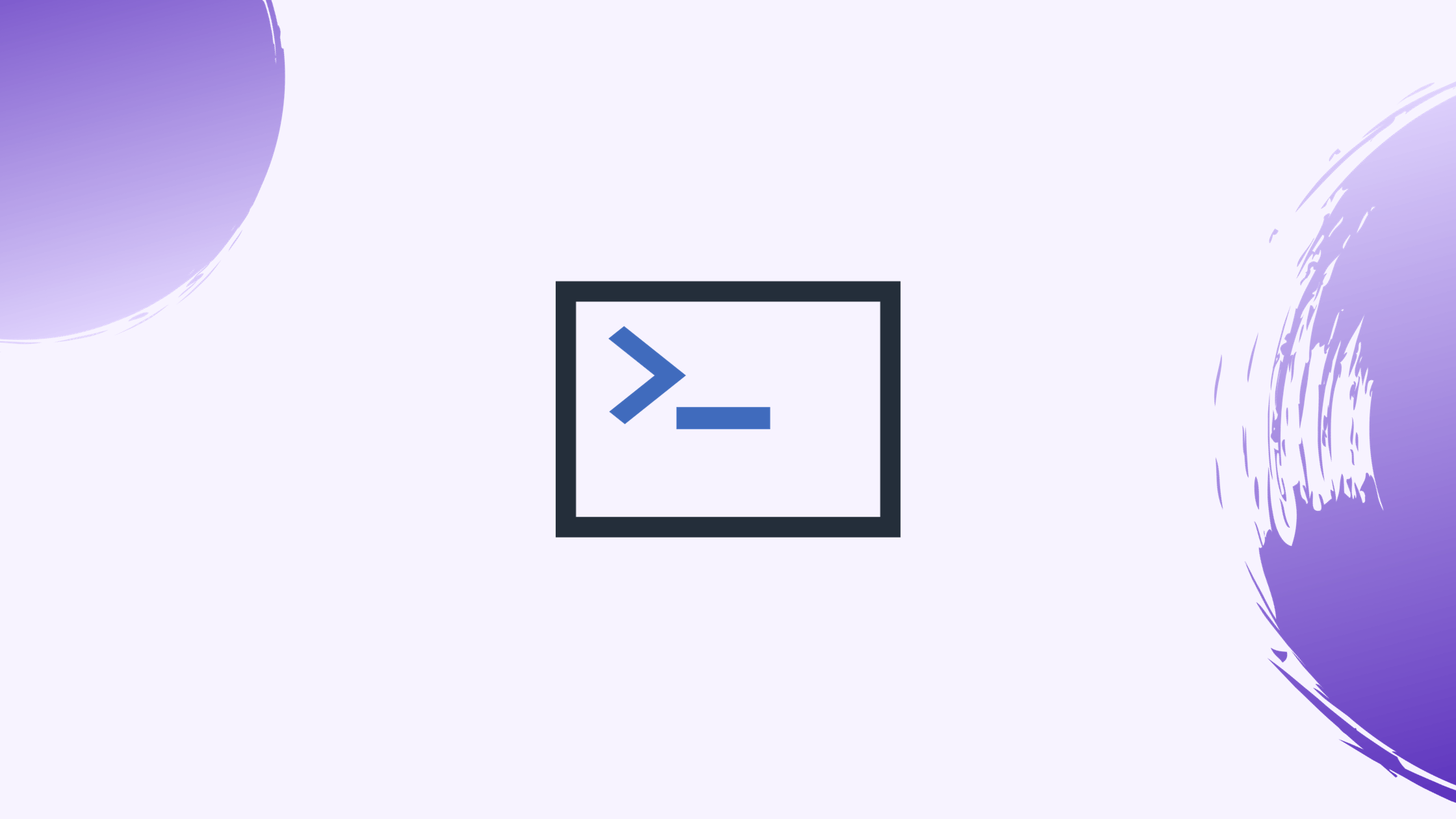
Introduction
Before we begin talking about how to increment and decrement variables in the Bash, let’s briefly understand - What does increment and decrement of variables mean?
Most common arithmetic operations when writing Bash scripts are incrementing and decrementing variables. These are most often useful for loops as a counter and can occur somewhere else in the script as well.
So, both incrementing and decrementing means adding or subtracting a value usually 1
, respectively, from a numeric variable. The arithmetic expansion is performed using double parentheses ((...))
and $((...))
or, with the built-in let
command. There are multiple ways to increment/decrement any variables.
In this tutorial, you will increment and decrement variable values in the Bash. We will also address some of the FAQs related to increment and decrement of variables.
Step 1 - Using + and - Operators
1) Given below is a most simple way to increment/decrement a variable. Do it by using the +
and -
operators.
i=$((i+1))
((i=i+1))
let "i=i+1"
i=$((i-1))
((i=i-1))
let "i=i-1"
This method will allow you to increment/decrement the variable by any value you want.
Below is an example of incrementing a variable with an until
loop:
i=0
until [ $i -gt 3 ]
do
echo i: $i
((i=i+1))
done
Output
i: 0
i: 1
i: 2
i: 3
Step 2 - Both += and -= Operators
1) In addition to basic operators explained above, bash also gives assignment operators +=
and -=
. These operators are useful to increment/decrement the value of the left operand with value specified after the operator:
((i+=1))
let "i+=1"
((i-=1))
let "i-=1"
2) You can decrement the value of i variable by 5
with while
loop as shown below:
i=20
while [ $i -ge 5 ]
do
echo Number: $i
let "i-=5"
done
Output
Number: 20
Number: 15
Number: 10
Number: 5
Step 3 - Using ++ and -- Operators
1) Both ++
and --
operators increment and decrement, respectively, it's operand by 1
and returns the value as follows:
((i++))
((++i))
let "i++"
let "++i"
((i--))
((--i))
let "i--"
let "--i"
2) The operators are useful before or after the operand. They are even known as:
- The prefix increment-
++i
- Prefix decrement-
--i
- Postfix increment-
i++
- and, Postfix decrement-
i--
The prefix operators firstly increment/decrement operators by 1
and after that return the new value of operators. On the other hand, postfix operators return the operator's value before it has been incremented or decremented.
There is no difference in using prefix/postfix operator if you only want to increment/decrement the variables. It will make a difference only when result of the operators is useful in some other operation or assignable to another variable.
The below examples demonstrate how ++
operator works. Especially when it is useful before and after its operant:
x=5
y=$((x++))
echo x: $x
echo y: $y
Output
x: 6
y: 5
x=5
y=$((++x))
echo x: $x
echo y: $y
Output
x: 6
y: 6
3) Below is an example on the way to use postfix incrementor in a bash script:
#!/bin/bash
i=0
while true; do
if [[ "$i" -gt 3 ]]; then
exit 1
fi
echo i: $i
((i++))
done
There is a disadvantage in using these operators. These variables can only be incremented or decremented by 1
.
FAQs to Increment and Decrement Variables in Bash
Can I increment/decrement variables within a loop in Bash?
Yes, incrementing/decrementing variables is often useful as loop counters in Bash scripts.
Are there any shorthand operators for incrementing/decrementing variables?
Yes, Bash provides shorthand operators like "++" for incrementing and "--" for decrementing variables.
How can incrementing and decrementing variables be helpful in loops?
Incrementing/decrementing variables act as counters, allowing loops to repeat a specific number of times or traverse through a range of values.
Is there a built-in limit on how many times a variable can be incremented or decremented?
No, there is no inherent limit, but it is essential to ensure the variable remains within the desired range.
Is incrementing/decrementing limited to numeric variables in Bash?
Yes, incrementing/decrementing in Bash is typically applied to numeric variables, not strings or other data types.
Are incrementing and decrementing variables used only in loops?
While commonly used in loops as counters, incrementing and decrementing variables can also be applied in other parts of a Bash script as needed.
What happens if I repeatedly increment a variable beyond its maximum value?
The variable will eventually wrap around to the minimum value and continue incrementing.
Conclusion
We hope this detailed guide helped you to increment and decrement variables in the Bash.
If you have any queries or doubts, please leave them in the comment below. We'll be happy to address them.