Introduction
Before we begin talking about how to read from stdin in Python, let's briefly understand – What is stdin?
stdin
in Python, refers to the standard input stream. It is a built-in file object that represents the input that is being read by a program. By default, the standard input stream is associated with the keyboard, meaning that any text typed in the console will be read from stdin
by the program.
Here, we have discussed three different ways to read data from stdin in Python-
- sys.stdin
- input() built-in function
- fileinput.input() function
In this tutorial, you will use read from stdin in Python. We will also address a few FAQs on how to read from stdin in Python.
1. Via sys.stdin to read from standard input
The interpreter uses the Python sys module stdin as standard input. It uses the input() function internally. A newline character (n) is inserted to the end of the input string. In order to remove it, use the rstrip() function. The following is a basic software that reads user messages from standard input and processes them. The user must type the "Exit" message in order for the software to stop.
import sys
for line in sys.stdin:
if 'Exit' == line.rstrip():
break
print(f'Processing Message from sys.stdin *****{line}*****')
print("Done")
Output:
Hi
Processing Message from sys.stdin *****Hi
*****
Hello
Processing Message from sys.stdin *****Hello
*****
Exit
Done
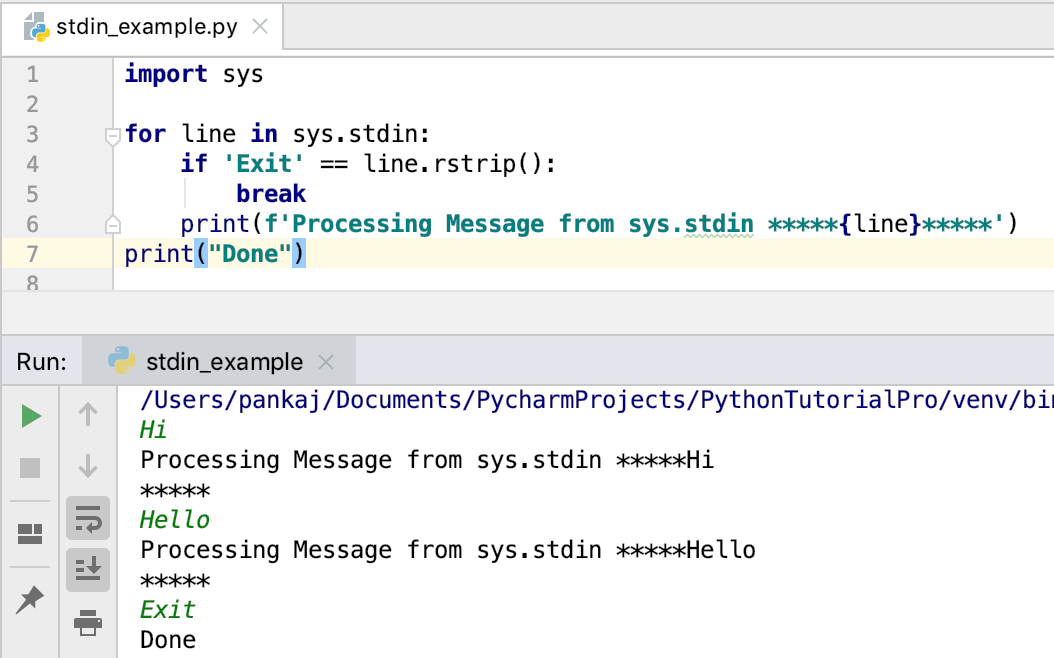
To check whether the user has input the "Exit" message or not, notice how rstrip() is used to remove the trailing newline character.
2. Via input() function to read stdin data
To read the data from standard input, we can use Python's input() function. Also, we have the option of sending the user a message. Here's a simple example of reading and processing the standard input message in an indefinite loop, until the user enters the Exit message.
while True:
data = input("Please enter the message:\n")
if 'Exit' == data:
break
print(f'Processing Message from input() *****{data}*****')
print("Done")
Output:
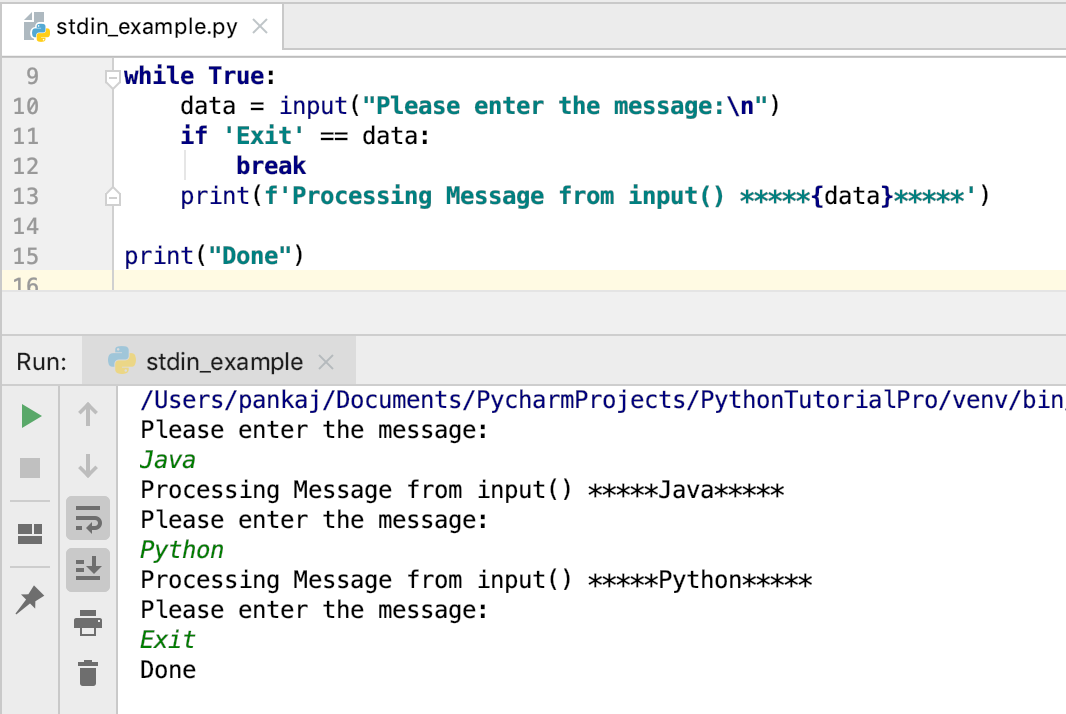
The user message is not appended with a newline by the input() function.
3. Reading Standard Input using fileinput module
The fileinput.input()
function can also be used to read from the standard input. The utility functions to loop through standard input or a list of files are provided by the fileinput module. The input() function reads arguments from the standard input when we don't pass it any arguments. Similar to sys.stdin, this function adds a newline character at the end of the input entered by the user.
import fileinput
for fileinput_line in fileinput.input():
if 'Exit' == fileinput_line.rstrip():
break
print(f'Processing Message from fileinput.input() *****{fileinput_line}*****')
print("Done")
Output:
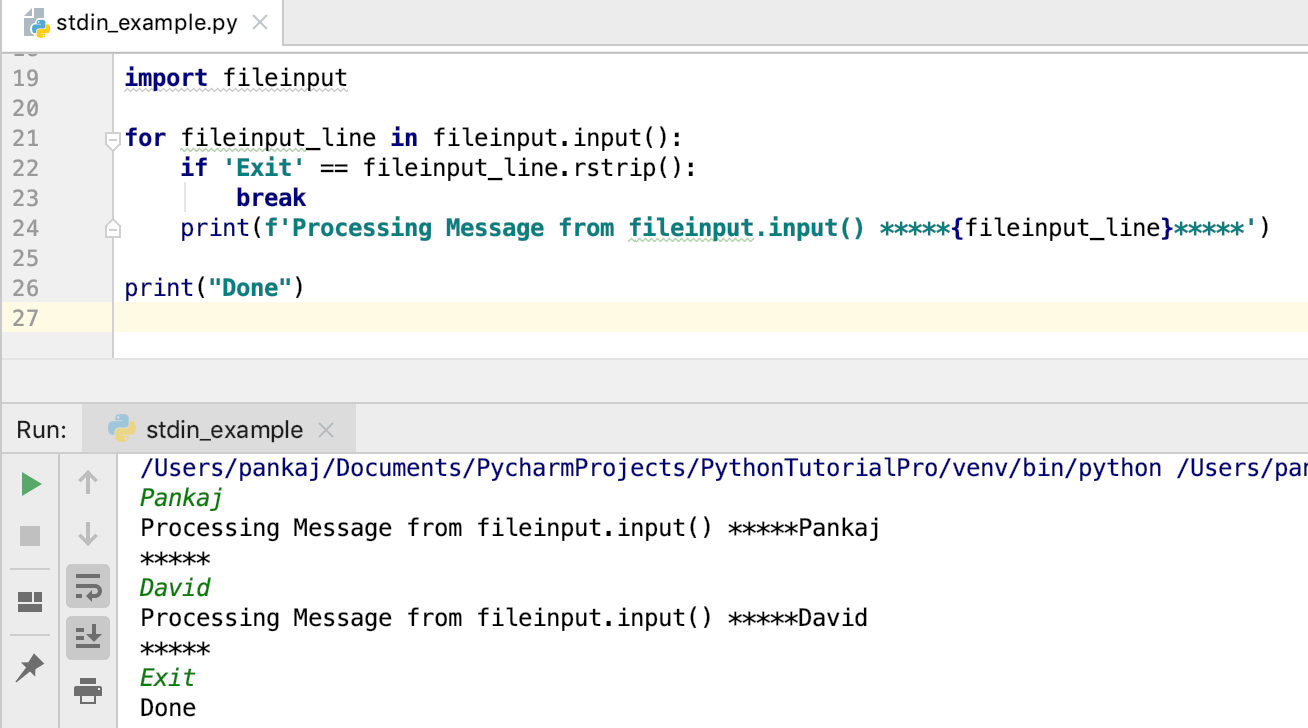
FAQs to Read From stdin in Python
What if I want to read only a specific number of characters from stdin?
You can use the sys.stdin.read(n)
method, where n
is the number of characters you want to read from stdin.
How can I read integers from stdin in Python?
You can use the int(input())
function to read integers from stdin. It will convert the input string to an integer.
Is there a way to handle end-of-file (EOF) while reading from stdin?
Yes, you can handle EOF by using a try-except block and catching the EOFError
exception that is raised when there is no more input to read.
How can I read input until a specific character or keyword is encountered?
You can read input until a specific character or keyword is encountered by using a loop and checking for the desired condition before continuing.
Can I read input from a file instead of stdin in Python?
Yes, you can read input from a file by opening it for reading and using fileObj.readline()
or fileObj.read()
to retrieve the content.
How do I handle input validation while reading from stdin?
You can validate user input by using conditional statements after reading input from stdin. You can check if the input meets certain criteria or falls within specific ranges.
What happens if I try to read input of the wrong type in Python?
Python will raise a ValueError
if you try to read input of the wrong type. You can handle this by using a try-except block to catch the exception and handle it gracefully.
Conclusion
In this tutorial, you learned how to read data from stdin in Python in three different ways: using sys.stdin, input() built-in function, fileinput.input() function. If you have any suggestions or queries, kindly leave them in the comments section.