Introduction
Before we discuss Python data types, let's first understand-What is Python ?
Python is a versatile programming language that supports a wide range of data types. Understanding the various data types is essential for effective programming and data manipulation in Python.
Python Data Types are used to specify a variable's type. We will list every data type and examine each one's functioning in this article. If you are just getting started with Python, do not forget to check out the Python tutorial for beginners first.
This tutorial provides a comprehensive list of Python data types and their key characteristics. We will also address a few FAQs on Python Data Types.
Python Data Types
Python supports a variety of data types. The following are some examples of built-in Python data types:
- Numeric data types: int, float, complex
- String data types: str
- Sequence types: list, tuple, range
- Binary types: bytes, bytearray, memoryview
- Mapping data type: dict
- Boolean type: bool
- Set data types: set, frozenset
1. Python Numeric Data Type
Python stores numerical values in a numeric data type, such as;
- int - a variable that stores signed integers of any length.
- long- contains long integers (exists in Python 2.x, deprecated in Python 3.x).
- float- contains floating precision numbers and is accurate to 15 decimal places.
- complex- contains complex numbers.
In contrast to C or C++, Python does not need us to define a datatype when we declare a variable. Values can be easily assigned to variables. But, we can use type() to check what kind of numerical value it is currently storing.
#create a variable with integer value.
a=100
print("The type of variable having value", a, " is ", type(a))
#create a variable with float value.
b=10.2345
print("The type of variable having value", b, " is ", type(b))
#create a variable with complex value.
c=100+3j
print("The type of variable having value", c, " is ", type(c))
If you run the above code, the output will look something like the image below.
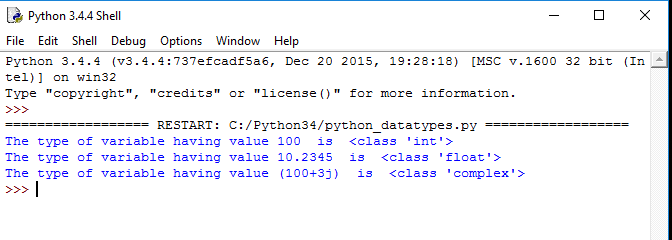
2. Python String Data Type
A sequence of characters make up the string. Unicode characters are supported by Python. Strings are often enclosed in single or double quotations.
a = "string in a double quote"
b= 'string in a single quote'
print(a)
print(b)
# using ',' to concatenate the two or several strings
print(a,"concatenated with",b)
#using '+' to concate the two or several strings
print(a+" concated with "+b)
The output from the code above looks like the image below:
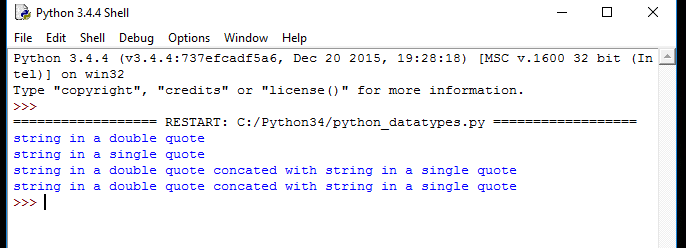
3. Python List Data Type
The list is a versatile data type that is only available in Python. In certain ways, it is similar to the array in C/C++. Nonetheless, the list in Python is significant because it can hold numerous types of data simultaneously. A list technically refers to an ordered group of data that is written with commas (,) and square brackets ([]).
#list of having only integers
a= [1,2,3,4,5,6]
print(a)
#list of having only strings
b=["hello","john","reese"]
print(b)
#list of having both integers and strings
c= ["hey","you",1,2,3,"go"]
print(c)
#index are 0 based. this will print a single character
print(c[1]) #this will print "you" in list c
The code above will result in output similar to this:
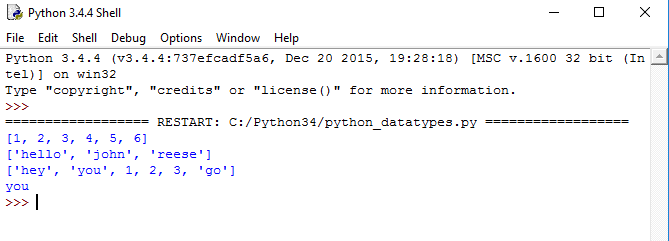
4. Python Tuple
A tuple, which resembles a list of data, is another form of data. But, it cannot be changed. This signifies that a tuple's data is write-protected. The data in a tuple is enclosed in parentheses and separated by commas.
#tuple having only integer type of data.
a=(1,2,3,4)
print(a) #prints the whole tuple
#tuple having multiple type of data.
b=("hello", 1,2,3,"go")
print(b) #prints the whole tuple
#index of tuples are also 0 based.
print(b[4]) #this prints a single element in a tuple, in this case "go"
This Python data type tuple example code will provide an output similar to the one shown below.
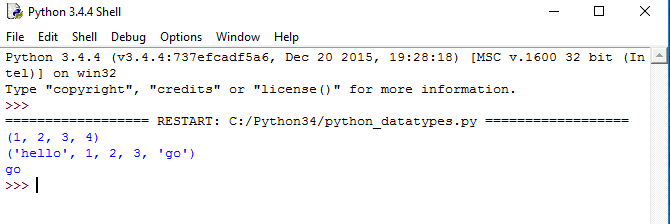
5. Python Dictionary
The Python dictionary is an unordered list of data in key-value pair format. It resembles the hash table type. Dictionaries are written in the format key:value
within curly braces. It is highly useful to obtain data from a vast amount of data in an optimal manner.
#a sample dictionary variable
a = {1:"first name",2:"last name", "age":33}
#print value having key=1
print(a[1])
#print value having key=2
print(a[2])
#print value having key="age"
print(a["age"])
The output of this Python dictionary data type example code will look like the image below.
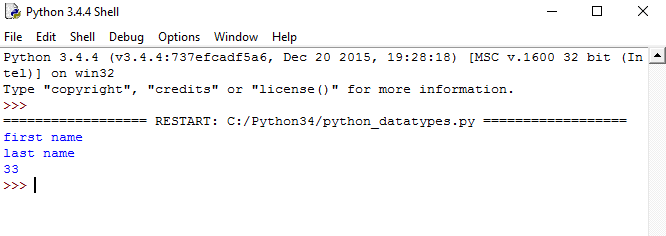
FAQs on Python Data Types
What is a data type in Python?
A data type in Python defines the category or classification of data and the operations that can be performed on it. It determines the behavior and characteristics of a variable or object.
What is the difference between mutable and immutable data types?
Mutable data types can be modified after creation, while immutable data types cannot. Examples of mutable types in Python include lists and dictionaries, while strings and tuples are immutable.
Can I create my own custom data types in Python?
Yes, Python supports object-oriented programming, allowing you to define and create your own custom data types using classes. This enables you to create objects with specific attributes and behaviors.
How do I determine the data type of variable or object in Python?
You can use the type()
function to determine the data type of variable or object in Python. For example, type(42)
will return <class 'int'>
.
Is Python a dynamically typed language?
Yes, Python is a dynamically typed language, which means that you do not need to declare the data type of a variable explicitly. The data type is inferred automatically based on the value assigned to the variable.
What are type hints in Python?
Type hints in Python allow you to specify the expected data type of variable or function parameter. Although not enforced by the interpreter, type hints serve as documentation and can be used by type-checking tools.
Can I convert between different data types in Python?
Yes, Python provides functions for converting between different data types. For example, you can use int()
to convert a value to an integer, float()
to convert it to a floating-point number, and str()
to convert it to a string.
Conclusion
This concludes our discussion of Python data types for today. Do not forget to execute each piece of code on your personal computer. Avoid simply copying and pasting. Make an effort to write the code lines on your own.
If you have any suggestions or queries, kindly leave them in the comments section.